- Review Sine Wave homework
- Thresholds and calibration review (lab)
- Arrays and for loops
- Control Multiple LEDs with ease using arrays and for loops(lab)
- Add movement with Motors (lab)
Thresholds and Calibration:
Most sensors do not generate a range of values from 0-1023 under normal working conditions. To accurately map a sensor you need to know the thresholds of the sensor. Each sensor will have a minimum and maximum threshold. Let's use a photocell to change the brightness of an LED. To do this first we will need to determine the minimum and maximum threshold of the photocell. Create the circuit below and then upload the code onto the Arduino or load the class3/autoCalibration sketch.
Once the program has been uploaded, look for the LED attached to pin 13 to light up. You now have 5 seconds to let the sensor take a hands free reading and also take a hands on reading. In the case of the photocell, let it be exposed to the ambient light of the room(this will serve as the maxThresh) and then cover up the sensor with your finger(this will serve as the minThresh). Once the pin 13 LED has turned off the loop() code will execute and you will see wonderful PWMing of a red LED.
Arrays and For Loops:
Arrays are a way of declaring multiple of the same type of variable in a row in memory. Usually they are used with the idea that the values are multiples of the same type of object. What I mean is while the value of the photocell is an integer it doesn't make sense to include it in an array of integers that represent the digital pins used to power LEDs. Arrays are declared a little differently then other variables. Here are a few ways to declare an array:
int myInts[6];// this creates an array that has 6 places but does not define what the values are
int myPins[] = {2, 4, 8, 3, 6};// this creates an array of 5 places and defines the values
int mySensVals[6] = {2, 4, -8, 3, 2};// this creates an array of 6 places but only defines the first 5 values
This is how you access the values that are store in the arrays above:
myPins[0], where the value would equal 2.
The number inside of the square brackets [ ] indicates which element to access. Arrays are 0 based, meaning the first element is considered the 0 place not the 1st place.
Here is how you change the value of an element of an array:
myPins[1] = 9;// now the values for myPins look like this: {2, 9, 8, 3, 6}
The real power of arrays is unleashed when combined with for loops. The structure of a for loop is a little confusing at first, but once you understand how to use them they are essential. Basically a for loop is a way of repeating a command a determined number of times. You can use an array to count from any number by any number to any number. This makes it perfect for working with arrays because instead of manually putting 0-4 inside of the [ ] to access the values of myPins array, you can use a variable.
Here is a for loop that prints the values of myPins array to the serial monitor:
for(int i = 0; i < 5; i++){
Serial.print(myPins[i]);
Serial.print(", ");
}
Serial.println();
This snippet of code will display the values with a comma and space between each value and then a line break after the last element has been printed. I recommend that you take a look at the for loop link above. The guys at Arduino have a wonderful description of how the mechanics work.
Let's put it into action so you can see how easy they are to use. Create the circuit below and then follow along in class to code it. I have included the code in class3/ledArray_switch. I will be coding this live in class so that you can see how to create such code. Feel free to follow along by typing or watching.
Here is the next circuit to create. This is almost the same circuit except we have switched out the switch for a potentiometer. We will use PWM on these LEDs based on a reading from the potentiometer.
Now let's swap out the potentiometer for the photocell.
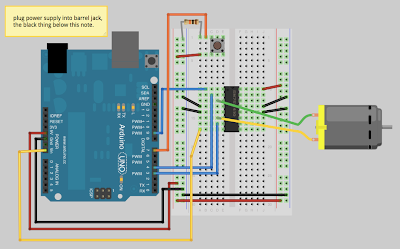
DO NOT RUN THIS CIRCUIT/SKETCH IF YOU DO NOT HAVE AN EXTERNAL POWER SUPPLY FOR THE MOTOR. Using only the 5v from the Arduino from USB is a great way to break your Arduino.Working with MotorsThe arduino can allow you to control much more than LEDs. In fact you can use the arduino to physically change your environment by using motors. There are many different types of motors, but the most common are brush DC motors, servo motors, and stepper motors.Brush DC motors : These are the most common motors. You will find them inside of toys, fans, blenders, printers, drills… the list goes on and on. The motor has two terminals, a positive and negative terminal. Simply apply power to one terminal and ground to the other and the motor will spin. Reverse which terminal gets power and ground and the direction reverses. An easy way to control the direction and power source of a motor is to use an H-Bridge.
This is how you wire your Arduino to an H-Bridge to a motor.
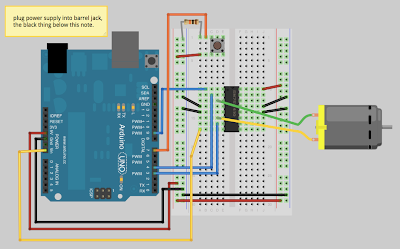
The code for running this circuit can be found here on the class pasteBin account.Stepper Motor : Stepper motors break down a full rotation into a number of steps. Rather than continually rotating like a DC motor, the stepper increments in steps or fractions of a rotation. While you can also use an H-Bridge to control a stepper it is easiest to use the EasyDriver stepper controller.Servo Motor : Servos are similar to Steppers, in that you tell it to go to a certain angle and it will stop at that angle. The difference is that most packages only have a rotational range of 180 degrees. Servos are used a lot in RC cars, boats and planes to control the steering mechanisms. At its heart a servo is a DC motor with circuitry to understand how far it has rotated. It also usually has gearing to increase its torque.Servos are incredible easy to use with the arduino. They have three wires, power, ground, and signal. A PWM signal is sent down the signal line and the servo uses that to determine where to rotate to. Arduino has a library that makes this all very easy for us. Build the circuit below and we will control the servo with a potentiometer.
I have been a herpes carrier for 2 years and I tried every possible means of curing but all of no useful until I saw a health promotion on a herbalist from West Africa who prepares herbal medicines to cure all sorts of diseases including #HSV and many others sickness but look at me today am very much happy and healthy, I'm telling you today don't lose hope keep trying God is with you, If you needs Dr.Chala help contact him on his email dr.chalaherbalhome@gmail.com or you can visit his website on http://drchalaherbalhome.godaddysites.com or https://mywa.link/dr.chalaherbalhome
ReplyDelete